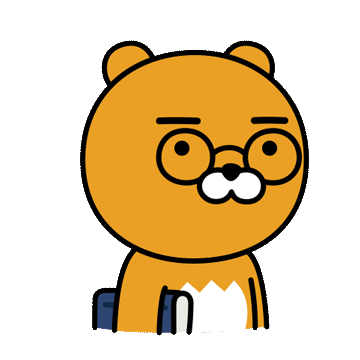
17장을 공부하다가.. this 에 대해서 조금 더 구체적으로 정리하고 싶어서 새로운 포스팅을 작성한담..
1. this 란 ?
함수나 메서드 내부에서 현재 실행되고 있는 컨텍스트(문맥)나 객체를 참조하는 역할을 한다.
하지만, this가 참조하는 대상은 그 사용 위치에 따라 달라지기 때문에 상황에 맞게 사용하면 된다!
📌바인딩이란?
식별자와 값을 연결하는 과정을 의미한다.
변수 선언은 변수 이름과 확보된 메모리 공간의 주소를 바인딩하는 것이었다.
this 바인딩은 this 와 this 가 가리킬 객체를 바인딩하는 것이다.
1. 전역 컨텍스트에서의 this
console.log(this);
// 전역 객체를 참조한다.
//브라우저에서는 `window`, Node.js에서는 `global`
2. 객체 메서드에서의 this
const person = {
name: 'hihilong',
greet: function() {
console.log(`Hello, my name is ${this.name}`);
}
};
person.greet(); // "Hello, my name is hihilong"
person.greet() 메서드에서 this는 person 객체를 참조한다.
그래서 this.name은 person 객체 내부의 있는 name이라는 프로퍼티와 같다.
3. 생성자 함수에서의 this
function Circle(radius) {
this.radius = radius;
this.getDiameter = function() {
return 2 * this.radius;
};
}
const circle1 = new Circle(5);
const circle2 = new Circle(10);
console.log(circle1.getDiameter()); // 10
console.log(circle2.getDiameter()); // 20
생성자 함수에서 this는 새로 생성된 인스턴스를 참조한다.
new 키워드를 사용해 Circle 생성자 함수를 호출하면, this는 각각의 인스턴스를 가리키게 된다.
circle1과 circle2는 각각 독립적인 인스턴스이며, 각 인스턴스는 자신의 radius 값을 가지고 있게 된다.
5. 화살표 함수에서의 this
const obj = {
name: 'hihilong',
greet: function() {
const innerGreet = () => {
console.log(`Hello, my name is ${this.name}`);
};
innerGreet();
}
};
obj.greet(); // "Hello, my name is hihilong"
화살표 함수는 자신만의 this 바인딩을 가지지 않고, 상위 스코프의 this를 그대로 사용하게된다.
여기서 innerGreet 함수는 obj의 this를 그대로 사용하여 obj 가 가지고 있는 프로퍼티인 this.name을 참조하게된다.
6. 메서드를 변수로 참조할 때의 this
const person = {
name: 'hihilong',
greet: function() {
console.log(`Hello, my name is ${this.name}`);
}
};
const greetFunction = person.greet;
greetFunction(); // undefined 또는 오류 (전역 객체의 `name`이 없기 때문에)
greetFunction을 독립적인 함수로 호출할 때, this는 person 객체를 참조하지 않게 되어,
undefined가 출력되거나 오류가 발생할 수 있다..
'Deep Dive 정리' 카테고리의 다른 글
[JS Deep Dive] 21장 - 빌트인객체 (0) | 2024.09.10 |
---|---|
[JS Deep Dive] 18장 - 함수와 일급객체 (0) | 2024.09.04 |
[JS Deep Dive] 17장 - 생성자 함수에 의한 객체 생성 (0) | 2024.09.04 |
[JS Deep Dive] 16장 - 프로퍼티 어트리뷰트 (0) | 2024.09.01 |
[JS Deep Dive] 15장 - let const 키워드와 블록레벨 스코프 (0) | 2024.08.28 |